About a month ago we made a rush trip through Shenzhen, Taipei, and Hong Kong, mainly to source components but also discussing with a few ODMs and EMSes. If you have never visited those places, they are each quite different, even though the are almost next to each other in terms of travelling time. In a couple of years, when the high speed rail from Kowloon to Shenzhen gets completed, Hong Kong and Shenzhen will also become closer to each other.
Anyway, we managed to squeeze some 15 meetings in four and a half days, at least if you count the breakfast meetings and dinners. Below I’m trying to give the flavour of how the life is down there.
Sunday arrival
After having slept good 6 hours and worked for almost 4 hours on slides in the Finnair crammed tourist class, jumping a few times over the lady sleeping next to me, we arrived to Hong Kong International Airport (HKIA) at around 1:40pm.
Following the instructions we were given, we proceeded to arrival hall E2 and bought ferry tickets from SkyPier to SheKou in Shenzhen. That turned out to be a mistake; it is much better to take a direct limousine from HKIA to the HaoTing (or maybe Futian checkpoint) cross border and cross the border there to the mainland China. We should have become suspicious when the teller told us that we need to buy first class tickets (at HK$30 extra each) as the tourist class was already full.

At the ferry we still didn’t have our baggage, as they are delivered only at ShuKou. Indeed, there was some package on the pier, behind a line, but we were still waiting. There were about 20 workers on the pier, waiting, too.
We waited, in the hot and humid weather, sweating. After some time, a crane lifted one metal container from the ferry. A couple of the workers helped the crane to take the container down, and bring it forward with a cart. An other set of guys emptied the container from baggage, then still others took it back to the pier end, the container was put aside from the cart. This all took maybe 3 minutes.
It did not stop there. There were 8 or 9 more containers that got the same handling, one by one. No pipelining. The crane stood still while the container was forwarded with the cart, emptied, took back, and put aside. With this 8-9 containers that whole process took well over 30 minutes.
Hence, it was well over 6pm when we finally managed to get our package, enter China, go though the customers, and get back out to the heat and humid air. Fortunately our “very good friend”, with his “very best friend” as a driver (for a driver he needed…), was waiting for us. They took us to the hotel. However, they managed also to give us some heartbeats as we first arrived at a very one-floor high and decades old Chinese motel… It turned out that the quite new and modern hotel was just at the other side of the block. A first experience of the modern Shenzhen street address system.
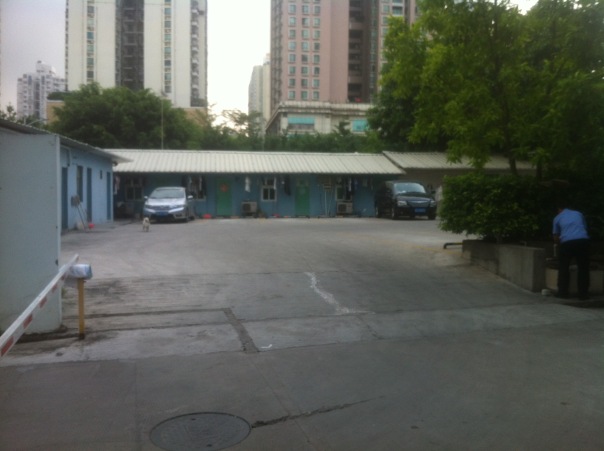
Sunday dinner
After our very good friends very good friend finding a parking place after some trouble and apparently also negotiation (the Shenzhen parking system is very interesting), we found more very good friends (that we of course had not met ever before) waiting for us in the restaurant.
There were a few interesting and perhaps instructional anecdotes during the dinner, clearly explaining how small scale business in China is different from business even in e.g. Taiwan. Firstly, the glass of my good old iPhone had been broken for some time. Hence, while it was already almost 10pm, my very good friend took my iPhone to prepare and reappeared an hour later, with a new cover glass and and (as it turned out later) a low quality protective film. (I don’t know yet if the cover glass is high quality or not. At least it had not broken yet. The previous one, exchanged in Finland, broke in three weeks, while the original one lasted well over two years.) The total cost was around $50 USD, including his taxi and the extra I gave that he could get a few more pijiu on the top of those he already had had.
As a second anecdote, once the best friends of my friend finally understood what we wanted, they called their good friend in that came now well after 10pm to do some business with us. Interestingly, one of the preceding communication problems was that in Mandarin there is no word that really means literally square. The word they usually use really means a square or a rectangle, i.e. any shape with four straight corners. There is a word that literally means “flat rectangle”, but apparently that can also be misunderstood. As far as I understood, the only way to say square is “not-long-rectangle-shaped [rectangle],” quite a long word.
Factory Monday
Our Monday was reserved for factory visits. We were picked up from our hotel by the sales manager of one of the Skyworth divisions. Their main business was tablet and comparable electronics product assembly, starting from the PCB assembly all the way to final testing and consumer packaging. It was quite interesting to see how Fordian and labor intensive this work was there. Real old style Fordian assembly lines mixed with some automated machines, but mostly manual work. In the factory we saw both the testing and assembly departments. I was also allowed to take some photos.
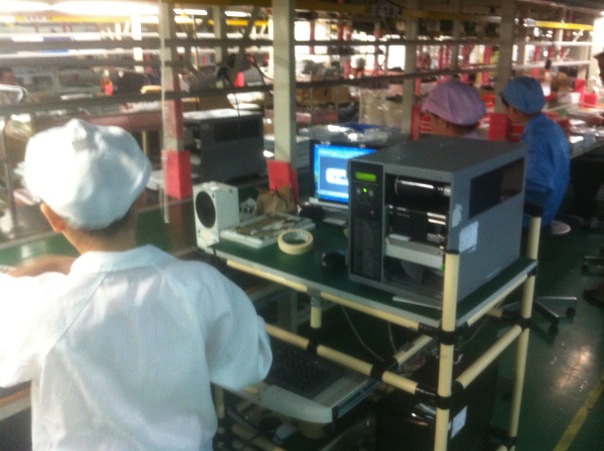
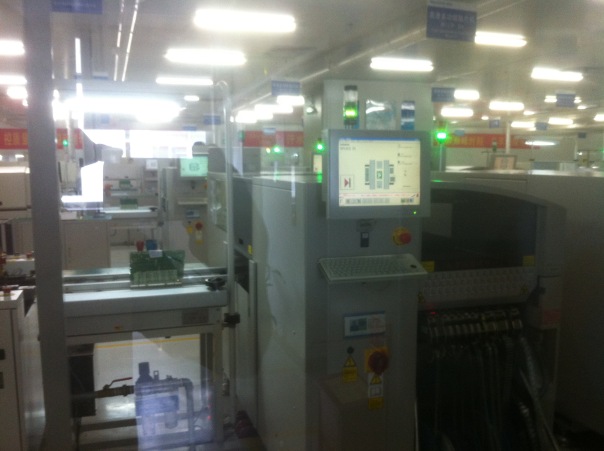
A driver for the second factory picked us up from the first factory’s gates and drove us to Dongguan, a city a couple of hours north from Shenzhen. In the style typical in China, our local host even arranged that the driver stopped by a small restaurant and bought us lunch on the way, as otherwise we would have missed our lunch. There was some traffic jam caused by road construction and therefore we arrived late, but apparently that was quite ok, this being China.
This second factory had a quite large clean room area. Even before we were allowed to their meeting rooms, we had to protect our shoes in order not to bring in dust even to the offices and meeting areas. I guess we were considered quite important potential customers, as we were taken into a boardroom-style large meeting room, where we gave our presentation and they gave theirs.
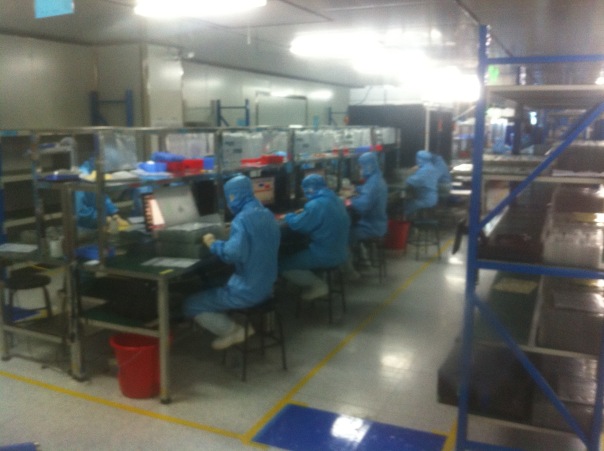
After the meeting we were taken to a tour to the factory, including a brief visit to one of the clean room areas. This was the first time I had a chance to experience wearing a basic clean room suite. Perhaps exciting the first time, but I bet it would become utterly boring quite soon.
A traffic jam
In Shenzhen area there are some quite interesting rules, such as that some cars are not allowed to be driven in the central Shenzhen (including the Futian area) during the evening rush hours; I didn’t get the exact hours but perhaps around five to eight or so. Consequently, the factory’s driver couldn’t take us back to Futian, but instead only to the metro station furthest away from the city. The jam off from the main highway to the metro station was quite tooty, the horns requiring space in an interesting disarray and the drivers more-or-less forcefully taking their space. It took us almost 30 minutes to get off from the highway, after which the remaining way to the metro didn’t take that long any more. According to locals, this was just a normal, everyday rush hour traffic jam.
Shenzhen metro
The Shenzhen metro is modern, easy, and nicely air conditioned. At all stations there are vending machines where you select your destination, pay (with cash), and get a green round smart token. Trips are cheap, just a few yuan, i.e. usually less than a euro. You can also buy a multi-trip smart card, but we didn’t try that. Buying the tokens turned out to be easy and cheap enough, especially as most of the people had the smart card and therefore we didn’t see any queues to the vending machines.

With the token at hand, you proceed to the entry gate. At most or maybe all stations you have to take your bags through X-ray, though to me this looked more like security theatre rather than real, people not passing their sometimes large hand bags through the X-rays without any objections by the guards.
The gates are like in any modern subway. You place the token over the sensor, the gates open, and you pass. At the destination, you drop the token to a slot. Not all gates accept the token on exit, though, so watch out. The cars are modern and nice, with space remaining in the evening when we made the longer trip, but quite crowded in the morning shortly before 9am, as most of the jobs start at 9am. Nothing compared to Tokyo, though. If you are planning to take the subway, it may be better to schedule your first meetings to start a little bit later.
Tuesday
On Tuesday morning we took the subway to the Science and Technology Park, to meet with the CTO of another Skyworth division. Compared to the people on Monday, he spoke very good English, making the communication uncomplicated. My feeling is that that the guy didn’t expect anything from the visit, mostly having agreed to meet with us only on courtesy. However, during the meeting he became clearly quite excited, seeing the potential how our offering could possibly change his business.
Shenzhen taxi
As our next meeting was only later, we took a taxi to Hua Qiang, the centre of the electronics “free” market in Shenzhen.
If you don’t speak Mandarin, the challenge of course is to tell where you are going. Having the address written in Chinese is the way, either on paper or on your cell phone. And even then be prepared to know the map and do the final few blocks on foot in the destination. At least in our case the taxies preferred to drop us somewhere close, at the street address, to figuring out all the way to the building we were going to.
HuaQiangBei electronics flea market
The Shenzhen electronics “free” or “flea” market is centred around the Hua Qiang metro station, mostly to the north (Bei) of it, hence the name HuaQiangBei, but also e.g. the display stores in the south (Nan).
You cannot really believe what the market is until you see it. It is absolutely huge. Most of the shops are really small, basically consisting of a counter and a teller or two, but there are also larger shops as well as Apple-store resembling stores by the there big and respected brands such as Huawei, Asus, etc.
To get an understanding of the magnitude, there are hundreds of these small shops focusing only on cell phone displays. There is one multi-storey department store sized building selling only used displays, apparently harvested from broken phones and refurbished. Next to it, there is another even larger building that sells new display components, mostly whole display modules for repairs, but also protective films, protective glasses, cover glasses, motherboards, and other stuff.

We found one shop that had a stack of iPhone schematics books, apparently for all iPhone models, at around $20 USD IIRC. When we asked them for the schematics of another not so usual phone, they offered us a USB stick that had schematics for most of the models for quite a few brands, at $60 USD. The sales girl didn’t know if it contained the schematics we wanted, but after a few phone calls an older guy came there. After some more Mandarin it became clear that unfortunately the stick didn’t contain the ones that we would have wanted.
Our guide was also able to find me a shop (among a few) where they sold bare, non-packaged LEDs. Just for fun, I bought an about 5 cm wide 30W LED, with enough of brightness to build a quite serious PoE light. I also bought a replacement battery for my laptop, from a slightly bigger and more serious shop, who also promised that the battery has a one year warranty. The price was less than half to what I paid for an Estonian battery that didn’t work.
Shenzhen street addresses
After some serious American coffee (Starbucks, of course, what else), it was the time to head back to our afternoon meeting. When trying to find this place, it became apparent that addresses in Shenzhen are almost as bad as in older Chinese cities. As you may know, the Chinese city planning is based on blocks. While in the Western Europe we think of a city as a set of crossing streets, with the houses along the streets, the Chinese think of a city as a set of districts, each district being divided into smaller roughly rectangular areas by main streets, with these smaller area being divided even smaller rectangles by smaller streets, etc, until you come to the level of what I would call very large macro blocks. These blocks still contain driveways and perhaps even what I would call streets, but these driveways do not necessarily have names, and even if they do, they are not necessarily included into the address. Instead, each building has a separate name.
BTW, the same address principle applies also to Hong Kong, even though to a smaller extent. For example, the address of my Hong Kong hotel was listed on the main street passing the block, where my hotel was located at a side alley in reality. You just need to know how the system works.
Our very best friends again
After our pre-planned meetings, we had a little bit of time left before we needed to head to HKIA for our Taipei flight. Our very good friends from the Sunday dinner had been trying to reach us all the time, and now they had a chance. As the timing was tight, they came to meet us at the hot and humid street next to our last meeting, from where we quickly moved to the air conditioned lobby instead.
In the standing 20 minutes meeting it became very clear that could possibly help us with our prototyping, through sourcing parts and schematics that had already turned out to be very hard to source. Almost needless to say that they never did.
Back to HKIA
By now we had learned that taking the ferry from ShuKou to HKIA would be potentially quite erratic. Following better advice, we had decided to take a limousine from Hao Ting, the car border crossing point near Futian Checkpoint. The way back to HKIA was very convenient, fast, and uneventful. While I’m a big fan of public transportation, this seems to be the fastest and most convenient way to get from HKIA to Shenzhen or vice versa, not counting taxi.
Today, to reach Shenzhen with HKIA Airport Express and metro would necessitate several exchanges and take more than two hours. Still, I would probably still favour airport express and metro over the ferry if I was going to Futian on a leisurely schedule, but the limo is clearly more convenient and faster. I’m really looking forward to the forthcoming fast rail connection from Kowloon to Shenzhen. However, before that gets completed, perhaps we should also consider flying directly to Shenzhen instead of HKIA, via Shanghai or Beijing. The flight route is almost the same, as the Hong Kong flights pass Beijing quite close.
Wednesday: meetings meetings
Our day in Taipei was full with very useful meetings. Also very American style efficient meetings, with some minor language problems. Some of the locals spoke excellent almost native level English, others less so but still good. The main problem was the accent, not the general level of English — for me the Chinese accent is quite hard to understand. I guess I would get better adjusted had I spent some more time there.
There were two minor difference compared to what I’ve typically experienced in the US or UK. Firstly, the companies brought quite big teams to meet us, even though these were just the initial meetings.
Taipei 101
While Maria had to leave for the airport, I still had one more day to go. Our hosts took me to Taipei 101, a skyscraper that was the worlds tallest building until 2010. While the skyscraper itself was interesting (and very very touristy), my inner engineer was impressed by the huge over 300 metric tons steel ball damper. Simplifying a little bit, the damper is a huge steel ball, painted in yellow and suspend in air by arm thick steel cables. Consequently, when the building bends due to typhoon winds or an earthquake, the ball will tend to stay in place due to its huge inertia. The bottom of the ball is connected to the building with huge hydraulic dampers, both in horizontal and diagonal directions. Hence, when the building moves and (initially) the ball doesn’t, it generates a huge force on the hydraulic dampers, compressing them on one side and extending on the opposite side.

Since there were no real engineering explanations on the operating principle, I still don’t quite know how the system really works. However, my guess is that the kinetic energy is converted into heat, thereby slowing down any vibrational forces the wind or quake causes. If the wind is steady, the building will still bend and the ball will follow, but if the wind is bursty, the dampers will slow down the movements. I would further guess that the dampers have been carefully designed to have their peak damping at the buildings natural frequencies, reducing the possibility that the whole building would start to resonate. With such a damper, the tall and narrow building would work as a huge rigid string or reed, attached in one end but free at the other. As any reed, it has its natural resonation frequency, where the vibrations would store more and more energy on each swing, until the building would break. With a tuned damper, this will not happen.
Thursday starting with HWTrek
My Thursday morning meeting was arranged by DK Lee and Jack Shen of HWTrek, an Taiwanese hardware accelerator. They have a new web platform where hardware startups can describe their projects. DK, Jack, and others at HWTrek will then try to connect them with the large number of experts they have in their database, creating connections for free. They also have a premium program, where you get even more help for a small fee. In the high end, they may act as your proxy in Taiwan, helping you to run your project with a local ODM or EMS.

Navigating in Hong Kong
After my remaining morning meetings in Taipei, I headed back to Hong Kong. My flight arrived at 2:20pm, in schedule. I had planned to take the Airport Express and metro to my next meeting, in Kowloon, being there at 4pm, which was almost exactly what I did, being there a few minutes past 4pm. Clearly this wasn’t China — I was able to plan like in Europe or US, and use public transportation like in any European city. Splendid.
BTW, it is worth noting that buying an Octopus card has been made unnecessarily complex. Firstly, you could buy it only using Hong Kong dollars, no credit cards accepted. You could well buy single and return Airport Express tickets with credit cards, but not Octopus cards. Secondly, in the the first place where you could buy train tickets, at the intermediary hall between the customs and the arrival hall, there was only a TravelEx ATM, blinking the red light of a bad exchange rate in my head. I headed to the arrivals hall. After some wondering I located an ATM, but surprise surprise, it turned out to be an other TravelEx ATM. However, this time it wasn’t the only one, but slightly behind it there were two Bank of China regular ATMs that accepted also international credit cards. I was able to make an easy cash advance from my debit card, meaning probably the best exchange rate possible.
The Airport Express is nice, fast, and comfortable. Quite similar to e.g. Paddington Express or Arlanda Express, with even free WiFi, IIRC.
Some Beijing duck
I had an afternoon meeting with one of the smaller EMSes. They are basically a family owned shop, with an R&D staff of a few tens and a factory nearby in China, employing a few hundred people. After a very nice meeting, I was given a royal treatment.

My hosts took me to Shangri La, which must be one of the best Chinese restaurants in Kowloon. There were six of us, I guess a good number in China (as apparently one of the guests was invited only to make the number even instead of odd). Me being not only a kind-of serial entrepreneur but also a former professor and a university fellow had made a strong impression on these people, they having made their homework properly. I guess people respect experience and academic credits much more in China (and Hong Kong) than in Europe or US, resembling more what it used to be also in Europe some 20–30 years ago.
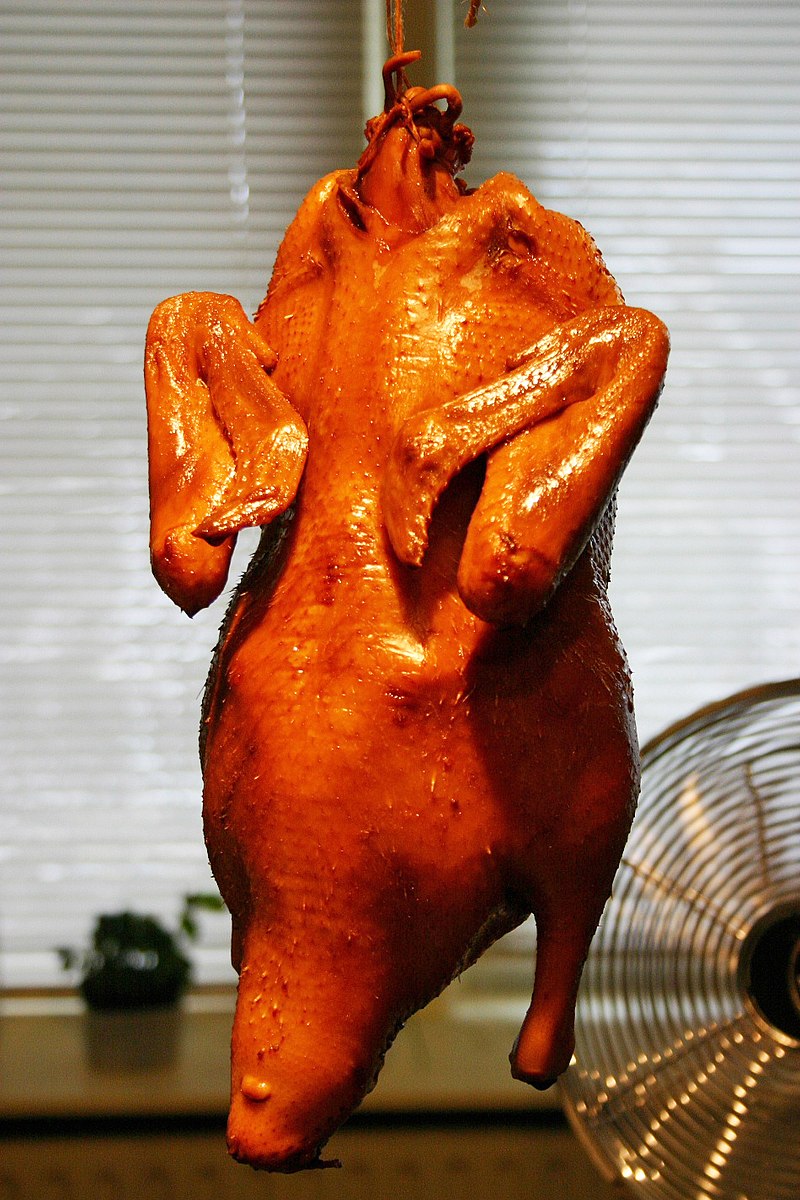
We had 北京烤鸭, Beijing [roasted] duck, which was good but dry. Shame the cook. Being an important person, I was made to select the wine, necessitating me to dig up all I managed to remember about Tuscany wines in order to be able to make a decent selection. The wine list was very long (mostly French wines of course), but it didn’t list the Chateaus or wine yards, not even the specific areas (like Chianti or Côte d’Odor), only the overall area (Tuscany, Burgundy, etc), and grapes. That made the selection hard. I turned to maître d’hôtel, who was quite knowledgable and was able to bring me two good but relatively young Chiati Classici to select from. As the wine was young (2012), it took quite some airing before the full body was revealed, initially giving a quite light expression. Partly my fault — I should have remembered this with young Chiantis, especially as I tasted it and as this is quite common, and thereby might have asked to exceptionally to decant this young wine to give it more air — but the maître could have know that too, given the class of the restaurant. Fortunately, we sat there in the restaurant for long enough that the wine managed to develop, being quite full bodied and lovely in the end.
Besides Beijing duck, we had thousand year eggs,spirit drowned king prawns, a small beluga that was really delicious, etc. An excellent dinner, apparently also testing my manners and ability to eat interesting food. If they ever visit us at Helsinki, we really need to take them to G.W. Sundmans, or why not Šašlik.
Friday: A nice coincidence
After a short night, I met a young man at the very quick breakfast I had time to eat before rushing to the airport.
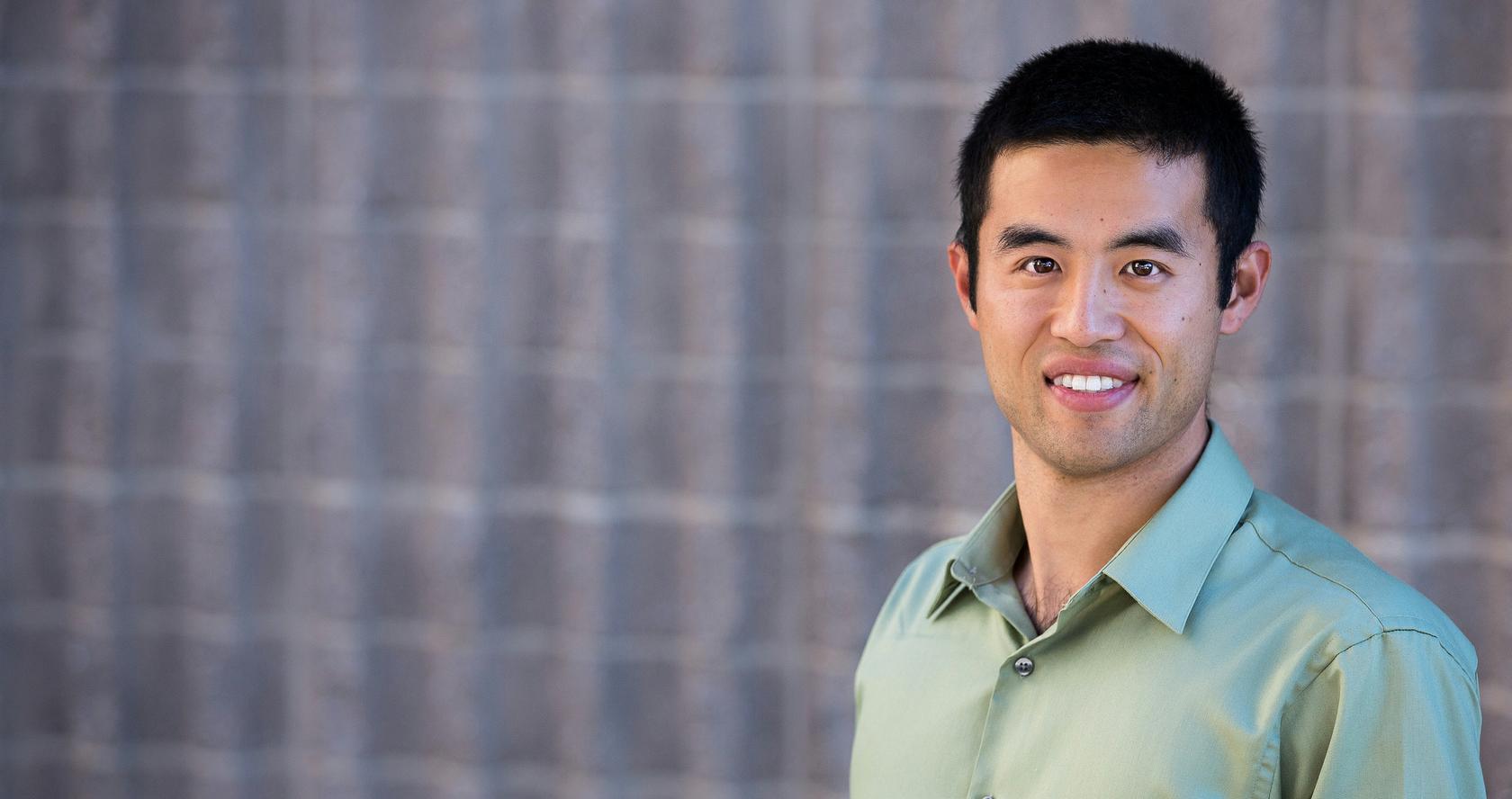
He turned out to be Bowei Gai, the founder of World startup report, nowadays trying to help the government of Indonesia in building a better Internet there. He turned out also to be a good friend of Benjamin Joffe of Haxelerator. Bowei finding me and our story interesting, we rushed out of the hotel together, walked briskly to the Hong Kong Airport Express station, and managed to get to the next train by last minute. Continuing to talk over the less than half an hour trip to the airport, we departed as we arrived at the airport.
I am really looking forward to visiting Hong Kong and Taipei again. Shenzhen wasn’t too bad either, even though the great Chinese firewall blocked me from my mail for two consecutive days. It is too bad that most probably I will not have time to return to the area for a while. Maybe I’ll manage to go there next time in the winter or spring, when the weather is not so humid and hot.
–Pekka